Chapter 25 - Digital Logic #
Digital logic is what it sounds like- it’s doing logical operations on digital data - 1’s and 0’s; True and False. Chain some of (er, a lot of) these logical operations together and you can do anything you want! Want to make your own CPU? Done. Want to make dedicated circuitry to do what your code does but hundreds of times faster? This is the way to do it.
As an example, here’s a rather artistic representation of a digital logic circuit that takes in a four bit binary number and outputs seven different signals corresponding to the lights on a 7-segment LED to show the right number (in hexadecimal)
Binary (0b) | Hex (0x) | Decimal |
---|---|---|
0000 | 0 | 0 |
0001 | 1 | 1 |
0010 | 2 | 2 |
0011 | 3 | 3 |
0100 | 4 | 4 |
0101 | 5 | 5 |
0110 | 6 | 6 |
0111 | 6 | 7 |
1000 | 8 | 8 |
1001 | 9 | 9 |
1010 | A | 10 |
1011 | B | 11 |
1100 | C | 12 |
1101 | D | 13 |
1110 | E | 14 |
1111 | F | 15 |
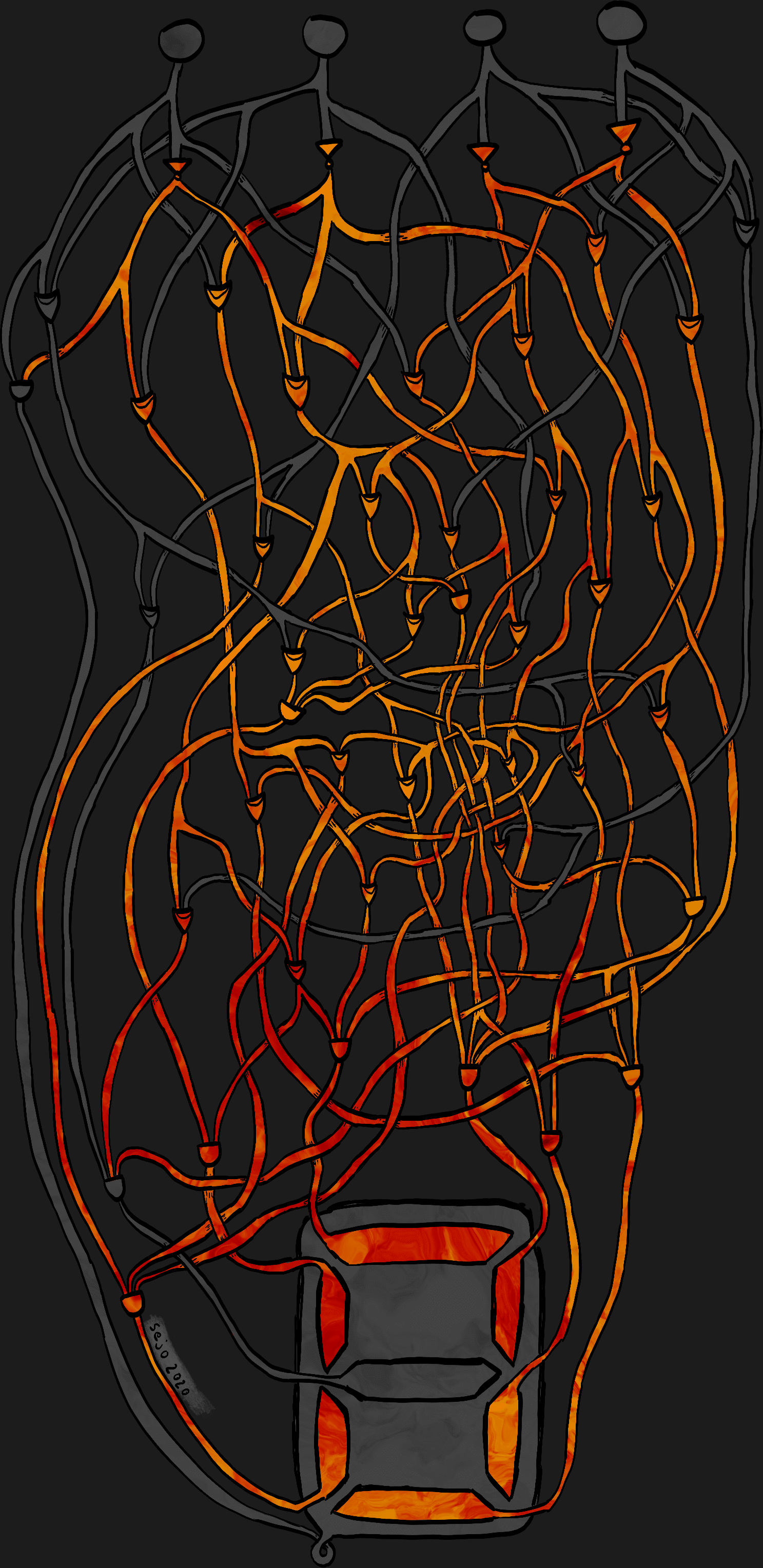
lineart by https://merveilles.town/@chirrolafupa, I (Vega) filled it in and turned it into a gif. To access the original line art, use a gemini browser to go to gemini://caracolito.mooo.com/coloring-computers/
Now, it’s worth considering here that this circuit is probably not the most efficient way to do this. This could also be done by just using a look up table (LUT), in that case, all of those individual logic elements are skipped and the input bits are basically just used as an address to look up a different value. The end result is the same. We’ll wrap back around to this idea later.
Some of this look familiar? If you’ve played Minecraft before, you may have gotten Redstone vibes from the GIF above. Those intuitions are correct. Many Redstone circuits are actually just clever ways of using the same binary logic ideas we’re about to go over!
What is digital logic? #
ignoring the analog world (10%/90%)
Truth Tables, K-Maps #
min/max terms, Don’t cares, SOP/POS, multiple out
[TODO] http://nandgame.com
Basic Logic Gates #
Not #
Image CC BY-SA 3.0, by Heron
Input | Output |
---|---|
0 | 1 |
1 | 0 |
[TODO] transistor implementation w/ CMOS, cost, power consumption at switch
AND #
True if A and B are true, else false. Often written as A∧B or just AB
Input A | Input B | Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
NAND (Not AND) #
False if A and B are true, else True. Often written as ¬(A∧B), ((¬A)∧(¬B)), or A⊼B
Input A | Input B | Output |
---|---|---|
0 | 0 | 1 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Bitwise AND
is often used in programming to make a mask of which bits you want to select from another value or to clear a specific bit or set of bits.
OR #
True if A or B are true, else False. Note, this includes if both are true. Often written as A∨B or A+B
Input A | Input B | Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Bitwise OR
is often used in programming to set a bit or set of bits without changing the other bits in the value.
NOR (Not OR) #
True if neither A or B are True, else False.
\( \text{Often written } \overline{x + y}\)Input A | Input B | Output |
---|---|---|
0 | 0 | 1 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 0 |
XOR (Exclusive OR) #
True if A or B are exclusively True, else False. Note, this does not include if both are true. Often written as A⊕B
Input A | Input B | Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
XNOR (Exclusive Not OR ) (also known as XAND) #
For two bits, most easily thought of as True if both bits are equal. More broadly, it is the negation of the exclusive or logic.
Image CC BY-SA 3.0, by Heron
Input A | Input B | Output |
---|---|---|
0 | 0 | 1 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Mux & Demux #
Go read the Multiplexer Wikipedia article. It’s actually excellent.
Encoders #
[TODO] like Binary to BCD
SOP & POS #
+ Kmaps
Basic combinational logic #
propagation delay, fan in/out, POS/POS
2’s Compliment, Floating Pt, Math #
overflow, carry, ripple, fast, mul, shift-and-add mult, divider,
Feedback Circuits #
Oscillators, Monostable, Osc if high, Bistable (controllable/not controllable)
Latches and Flip Flops #
SR, Dl, edge triggering, Dff, Tff, JKff,
Registers #
+ Shift registers
Clocking & Counters #
[TODO] dividers, multipliers, delay
Memory Circuits #
Static Memory #
Dynamic Memory #
https://www.adafruit.com/product/1895
Making Some Circuits #
There’s a ton of simulators out there for making simple circuits educationally, but most sorta suck, so I’m going to delve right into something fun: Minecraft
If you don’t own Minecraft and can’t afford it, MineTest with mesecons is an option. It’s… not great. (sorry MineTest team, I still love what you’re doing <3 ) But it is Open Source. If you’re using Arch Linux (and updates haven’t broken things since writing) you should be able to install
MineTest
from the community repo andmesecons-git
from the AUR.
Half Adder #
Full Adder #
Analog To Digital & Digital To Analog #
Schmitt Triggers #
I like to think of a schmitt trigger as the logical equivalent of a heavy light switch. Once it’s on, it takes a fair amount of force to turn off, and once it’s off, it takes a fair amount of force to turn on, but in our case that force is a voltage.
This means we can say “Until you see 1V at the input, don’t turn on” and “Until you see -1V at the input don’t turn off”. If after being turned on, it went to .5, or even -.5V, it would stay on. If it was off and we gave it input of .5V, it would stay off. We have to cross the threshold either way to change state.
Of course, we can make schmitt triggers with different thresholds and output levels.
Image by Alessio Damato - CC BY-SA 3.0. From Wikipedia.
ADCs #
[TODO] + Parallel Output vs via data bus
DACs #
More Digital Logic resources #
We’ll come back to look at more complex digital logic in chapter 28 “Let’s try out programmable logic” and 29 “Let’s make our own CPU” , but if you’re hungry for even more, here are some resources